21x faster than Express
Supercharged by Bun runtime, Static Code Analysis, and Dynamic Code Injection
Being one of the top-performing TypeScript frameworks. Comparable to Go and Rust.
Elysia Bun
2,454,631 req/sSwoole PHP
1,035,418Gin Go
676,019Spring Java
506,087FastAPI PyPy
448,130Fastify Node
415,600Express Node
113,117Nest Node
105,064
Measure in requests/second. Result from official TechEmpower Benchmark Round 22 (2023-10-17) in PlainText.
Made for Humans
Focus on productivity ++
If you found yourself writing code for the framework, then there's something wrong with the framework.
That's why Elysia invests time to experiment with design decisions to craft the most ergonomic way possible for everyone
From built-in strict-type validation to a unified type system, and documentation generation, making an ideal framework for building servers with TypeScript.
import { Elysia } from 'elysia'
new Elysia()
.get('/', 'Hello World')
.get('/json', {
hello: 'world'
})
.get('/id/:id', ({ params: { id } }) => id)
.listen(3000)
Just Value
No need for an additional method, just return the value to send data back to the client.
Whether it's a regular string, or complex JSON, just return the value and Elysia will handle the rest
import { Elysia, t } from 'elysia'
new Elysia()
.post(
'/profile',
// ↓ hover me ↓
({ body }) => body,
{
body: t.Object({
username: t.String()
})
}
)
.listen(3000)
Type Safety
Powered by TypeBox, Elysia enforces type-strict validation to ensure type integrity by default
Elysia infers types to TypeScript automatically to create unified type system like statically typed language
import { Elysia, t } from 'elysia'
import { swagger } from '@elysiajs/swagger'
import { users, feed } from './controllers'
new Elysia()
.use(swagger())
.use(users)
.use(feed)
.listen(3000)
OpenAPI / Swagger
Elysia generates OpenAPI 3.0 specs automatically to integrate with various tools across multiple languages
Thanks to OpenAPI compliance, Elysia can generate Swagger in one line with the Swagger plugin.
End–to-End Type Safety
Synchronize types across all applications.
Move fast and break nothing like tRPC.
Hover code below to see type definition
// server.ts
import { Elysia, t } from 'elysia'
const app = new Elysia()
.patch(
'/user/profile',
({ body, error }) => {
if(body.age < 18)
return error(400, "Oh no")
if(body.name === 'Nagisa')
return error(418)
return body
},
{
body: t.Object({
name: t.String(),
age: t.Number()
})
}
)
.listen(80)
export type App = typeof app
// client.ts
import { treaty } from '@elysiajs/eden'
import type { App } from './server'
const api = treaty<App>('localhost')
const { data, error } = await api.user.profile.patch({
name: 'saltyaom',
age: '21'})
if(error)
switch(error.status) {
case 400:
throw error.value
case 418:
throw error.value
}
data
It works with that
Being one of the most popular choices for a Bun web framework, likely there is a plugin for what you want.
If the plugin you need is not there, it's easy to create one and share it with the community.
Can't find what you're looking for?
Join the community
Elysia is one of the biggest communities for Bun first web frameworks.
You can ask your questions / propose a new feature / file a bug with our community and mainters.
Made possible by you
Elysia is not backed by any organization
Made possible by the support of the community and you
Scalar
for 4 months
Jarred Sumner
for 7 months
_typedev
for 7 months
DOM CHAROENYOS
for 3 months
mabujaber
for 2 months
Naoki Takahashi
for 11 days
Christian Rishøj
for 5 months
henrycunh
for 3 months
Khyber Sen
for 3 months
MeCode: Software Crafting Studio
for 3 months
yoyismee.eth
for 3 months
Augusto Chaves
for 3 months
Nemanja
for 2 months
Hassadee Pimsuwan
for 2 months
CellaJS
for a month
kubilay
for 17 days
d3or
for 3 months
Thanatat Tamtan
for 2 years
Jake Gibson
for a year
xHomu
for 8 months
Marco Beier
for 3 months
Worrapop Stk
for 2 months
Ronald Dijks
for 19 days
Patrick Wozniak
for 9 months
Jittat Fakcharoenphol
for 8 months
Kevin Haupt-Müller
for 7 months
Ajit Krishna
for 7 months
David L. Bowman
for 3 months
Ciro Spaciari
for 3 months
Kamil Jakubus
for 3 months
Lucca Tisser Paradeda
for 2 months
✦ freddie
for 2 months
Kira Kitsune
for a month
Ricardo Devis Agullo
for a month
Foxie Solutions
for a month
Snorre Magnus Davøen
for 25 days
4ndrs
for 21 days
Elikem Medehou
for 17 days
ga1az
for 17 days
smilegod
for 2 months
Nutthapat Pongtanyavichai
for 2 years
Manassarn "Noom" Manoonchai
for 2 years
Phawit Pornwattanakul
for 2 years
Vorrapong Kertnat
for a year
henaff
for 7 months
First Sutham
for 7 months
Thitipong Tapianthong
for 5 months
stanley
for 3 months
Amju
for 3 months
ASPeXP
for 3 months
Light Yagami
for 3 months
Dominik Seger
for 3 months
Kaze
for 3 months
Amer Alimanović
for 3 months
Marcello
for 2 months
Nayuki
for a month
Altin Thaci
for a month
Wutthipong Luangsanam
for a month
Kittichai Raksawong
for 25 days
白田 連大
for 14 days
Lyor
for 13 days
And you
Become sponsor
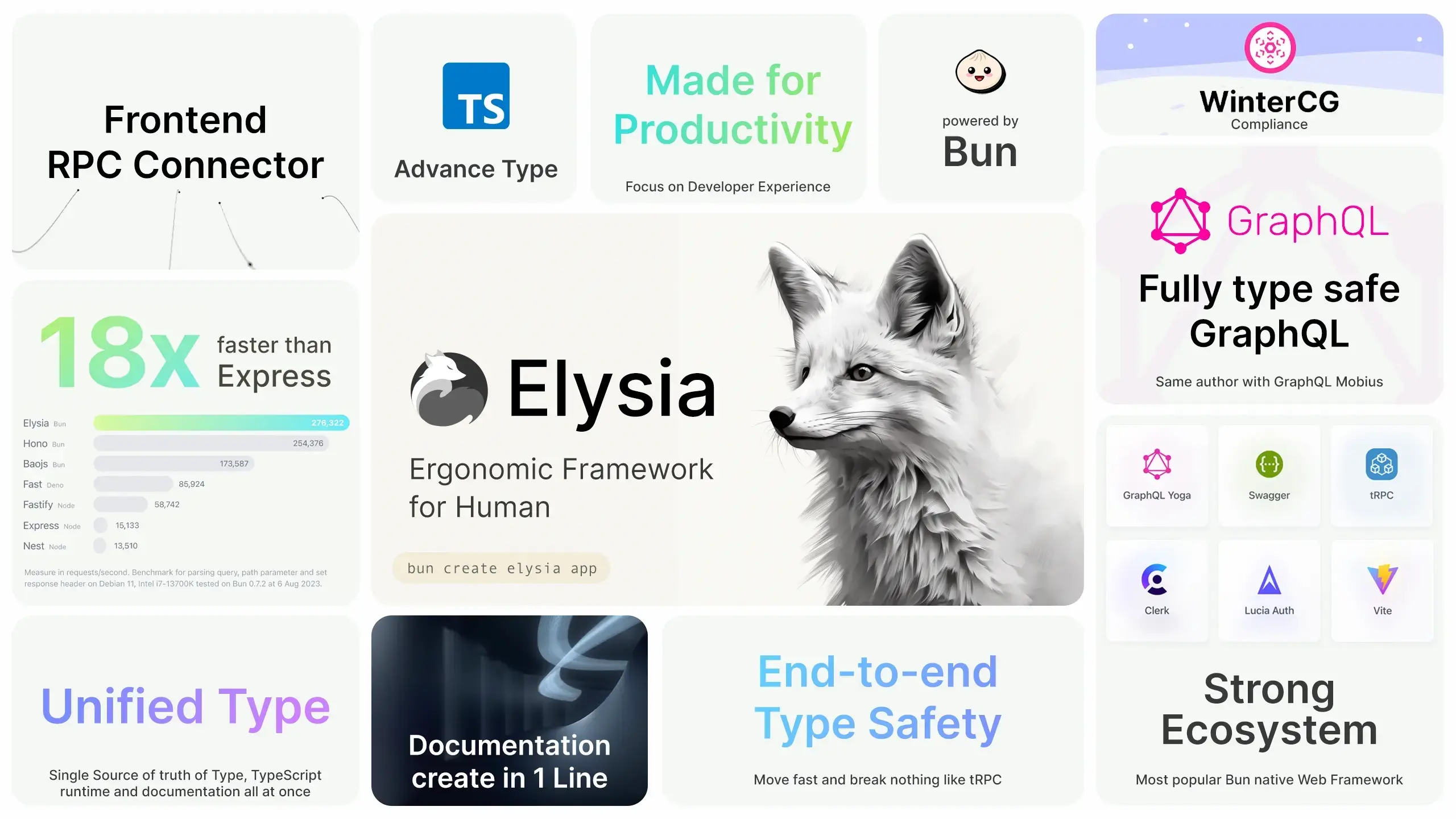
Start in minutes
Scaffold your project, and run server in no time
bun create elysia app