Swagger Plugin
This plugin generates a Swagger endpoint for an Elysia server
Install with:
bun add @elysiajs/swagger
Then use it:
import { Elysia } from 'elysia'
import { swagger } from '@elysiajs/swagger'
new Elysia()
.use(swagger())
.get('/', () => 'hi')
.post('/hello', () => 'world')
.listen(3000)
Accessing /swagger
would show you a Scalar UI with the generated endpoint documentation from the Elysia server. You can also access the raw OpenAPI spec at /swagger/json
.
Config
Below is a config which is accepted by the plugin
provider
@default scalar
UI Provider for documentation. Default to Scalar.
scalar
Configuration for customizing Scalar.
Please refer to the Scalar config
swagger
Configuration for customizing Swagger.
Please refer to the Swagger specification.
excludeStaticFile
@default true
Determine if Swagger should exclude static files.
path
@default /swagger
Endpoint to expose Swagger
exclude
Paths to exclude from Swagger documentation.
Value can be one of the following:
- string
- RegExp
- Array<string | RegExp>
Pattern
Below you can find the common patterns to use the plugin.
Change Swagger Endpoint
You can change the swagger endpoint by setting path in the plugin config.
import { Elysia } from 'elysia'
import { swagger } from '@elysiajs/swagger'
new Elysia()
.use(
swagger({
path: '/v2/swagger'
})
)
.listen(3000)
Customize Swagger info
import { Elysia } from 'elysia'
import { swagger } from '@elysiajs/swagger'
new Elysia()
.use(
swagger({
documentation: {
info: {
title: 'Elysia Documentation',
version: '1.0.0'
}
}
})
)
.listen(3000)
Using Tags
Elysia can separate the endpoints into groups by using the Swaggers tag system
Firstly define the available tags in the swagger config object
app.use(
swagger({
documentation: {
tags: [
{ name: 'App', description: 'General endpoints' },
{ name: 'Auth', description: 'Authentication endpoints' }
]
}
})
)
Then use the details property of the endpoint configuration section to assign that endpoint to the group
app.get('/', () => 'Hello Elysia', {
detail: {
tags: ['App']
}
})
app.group('/auth', (app) =>
app.post(
'/sign-up',
async ({ body }) =>
db.user.create({
data: body,
select: {
id: true,
username: true
}
}),
{
detail: {
tags: ['Auth']
}
}
)
)
Which will produce a swagger page like the following
Security Configuration
To secure your API endpoints, you can define security schemes in the Swagger configuration. The example below demonstrates how to use Bearer Authentication (JWT) to protect your endpoints:
app.use(
swagger({
documentation: {
components: {
securitySchemes: {
bearerAuth: {
type: 'http',
scheme: 'bearer',
bearerFormat: 'JWT'
}
}
}
}
})
)
export const addressController = new Elysia({
prefix: '/address',
detail: {
tags: ['Address'],
security: [
{
bearerAuth: []
}
]
}
})
This configuration ensures that all endpoints under the /address
prefix require a valid JWT token for access.
Contributors
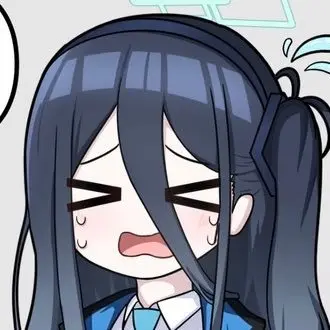


